Customization
Custom type formatting
It’s beneficial to be able to convert your custom types to a human readable string.
If your type doesn’t match any of the supported types, it will default to the "?"
output, which isn’t very helpful.
All that is needed it to make a function available for the template matching to find it.
Here is an example that takes a custom enum and converts that to a readable output:
#include <stdint.h>
#define JC_TEST_IMPLEMENTATION
#include <jc_test.h>
enum Result { RESULT_OK, RESULT_FAIL };
static const char* ResultToString(Result r) {
switch(r) {
case RESULT_OK: return "RESULT_OK";
case RESULT_FAIL: return "RESULT_FAIL";
}
}
template <> char* jc_test_print_value(char* buffer, size_t buffer_len, Result r) {
return buffer + JC_TEST_SNPRINTF(buffer, buffer_len, "%s", ResultToString(r));
}
static Result ReturnFail() {
return RESULT_FAIL;
}
TEST(Example, CustomPrint) {
ASSERT_EQ(RESULT_OK, ReturnFail());
}
int main(int argc, char **argv)
{
jc_test_init(&argc, argv);
return jc_test_run_all();
}
# Compile the app
$ clang++ -I src hugo/static/code/example_custom_print.cpp
# Run the app
$ ./a.out
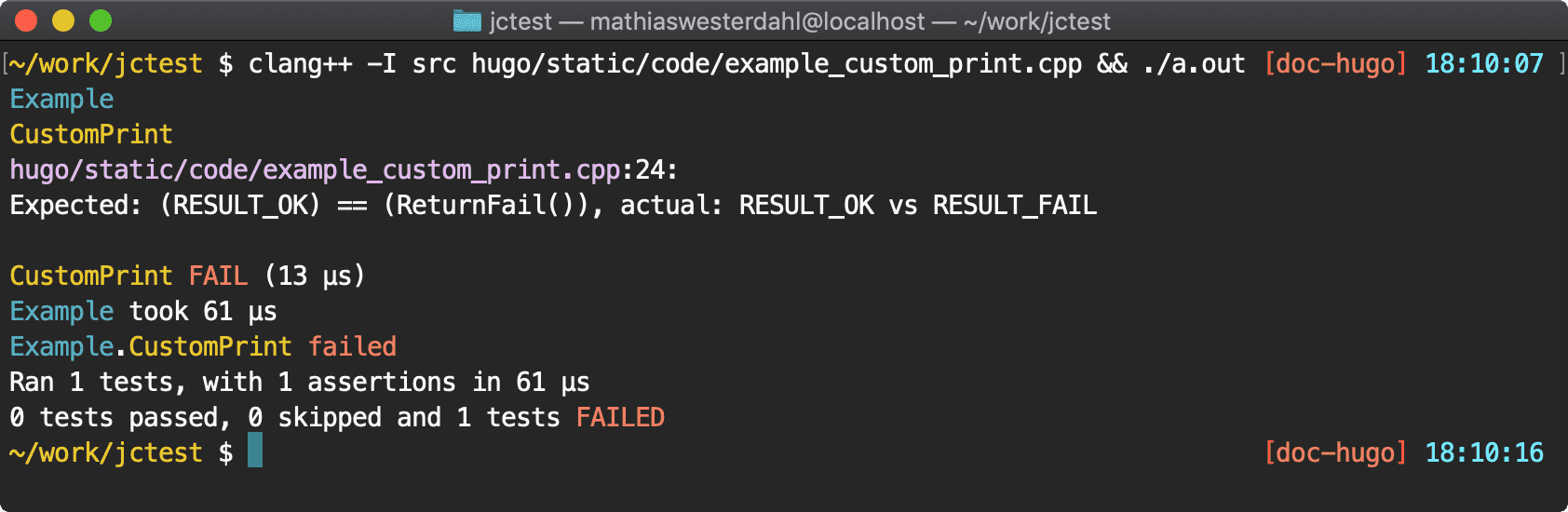